How Developers Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
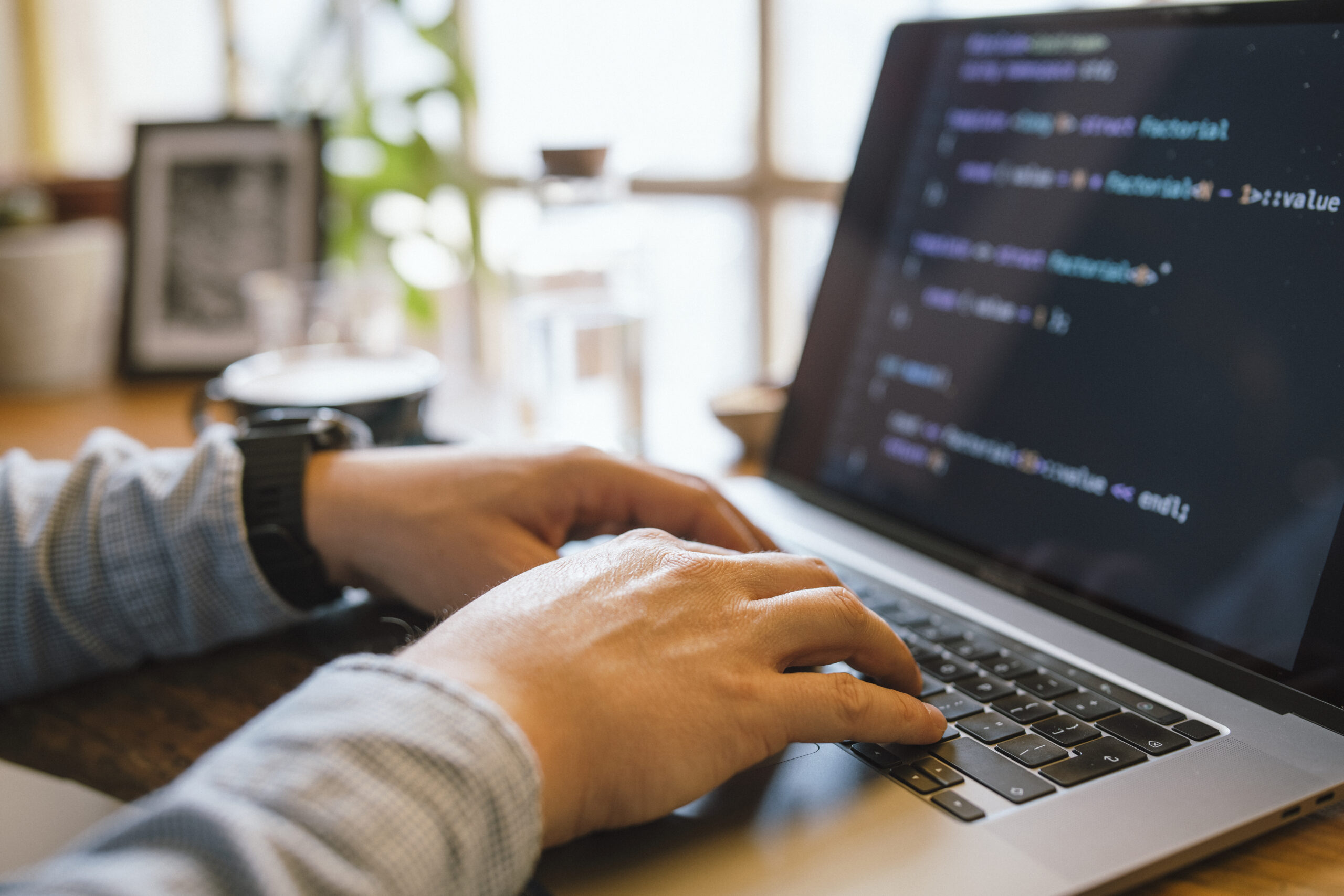
Debugging is One of the more essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are a number of strategies to help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is 1 Element of progress, being aware of the best way to interact with it correctly for the duration of execution is equally vital. Present day advancement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these tools can perform.
Consider, for example, an Built-in Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, step by way of code line by line, as well as modify code to the fly. When employed the right way, they Allow you to notice specifically how your code behaves all through execution, which can be a must have for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn into snug with version Manage techniques like Git to be aware of code record, find the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when concerns come up, you’re not misplaced at midnight. The higher you understand your resources, the more time you are able to invest solving the actual problem rather than fumbling as a result of the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Inquire questions like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The more element you might have, the less difficult it turns into to isolate the precise problems below which the bug takes place.
As soon as you’ve collected plenty of details, try to recreate the situation in your local natural environment. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply help expose the challenge but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But once you can regularly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra effectively, test possible fixes safely, and communicate much more clearly together with your team or end users. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and learning to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts through progress, Data for basic occasions (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. Too much logging can obscure vital messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continuously. Incorporate context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what circumstances are achieved, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments wherever stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-imagined-out logging solution, you'll be able check here to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective fixing a secret. This mentality helps break down complicated concerns into workable sections and observe clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as you may devoid of leaping to conclusions. Use logs, examination situations, and consumer stories to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What can be producing this actions? Have any improvements just lately been created for the codebase? Has this problem happened right before underneath equivalent situations? The goal should be to slim down prospects and determine potential culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a managed surroundings. In the event you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome guide you closer to the reality.
Shell out close awareness to tiny details. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface afterwards.
And lastly, keep notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated programs.
Generate Tests
Composing assessments is among the most effective methods to increase your debugging techniques and In general improvement efficiency. Exams not merely enable capture bugs early but additionally serve as a safety Internet that provides you self confidence when building variations to your codebase. A well-tested application is easier to debug since it permits you to pinpoint just wherever and when a challenge happens.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you quickly know in which to search, considerably reducing some time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-conclusion assessments into your workflow. These assist ensure that several areas of your application do the job collectively smoothly. They’re significantly valuable for catching bugs that happen in complex devices with several factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what problems.
Creating assessments also forces you to Assume critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with By natural means potential customers to higher code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a annoying guessing video game right into a structured and predictable procedure—aiding you capture additional bugs, faster and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the situation—gazing your screen for hours, attempting Remedy soon after Resolution. But One of the more underrated debugging applications is solely stepping absent. Having breaks helps you reset your mind, reduce aggravation, and often see the issue from a new perspective.
If you're too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short walk, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a very good rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it essentially leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your brain Place to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you a little something beneficial should you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in the workflow or understanding and help you build much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital parts of your progress journey. In any case, a lot of the greatest builders usually are not those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.